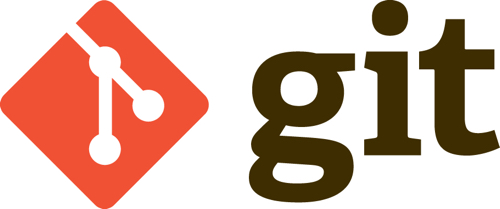
If you want to use multiple GitHub accounts with SSH keys on your computer, you have to adapt your ssh- and git-configuration.
In this tutorial I’ll explain the configuration steps for the following scenario:
GitHub user 1
- username: user01
- name: John Doe
- email: john.doe@abc.com
- repository: git@github.com:user01/testrepo01.git
GitHub user 2
- username: user02
- name: John Doe
- email: jd@work.com
- repository: git@github.com:user02/testrepo02.git
1. Create SSH keys for your accounts
$ ssh-keygen -t rsa -b 4096 -C "id_rsa_github_user01" -f ~/.ssh/id_rsa_github_user01
$ ssh-keygen -t rsa -b 4096 -C "id_rsa_github_user02" -f ~/.ssh/id_rsa_github_user02
When you create your ssh keys you can decide, wether you encrypt your private keys with a passphrase or not.
TIP: The purpose of the passphrase is to encrypt your private key. This makes the key file by itself useless to any attackers.
Now you should have the following files:
- ~/.ssh/id_rsa_github_user01
- ~/.ssh/id_rsa_github_user01.pub
- ~/.ssh/id_rsa_github_user02
- ~/.ssh/id_rsa_github_user02.pub
2. Add your SSH keys to the agent
To automatically load the keys into the ssh-agent and to store your passphrases in the keychain, add the following code to your ~/.ssh/config
.
# global
Host *
UseKeychain yes
AddKeysToAgent yes
Now you have to add your keys to the ssh agent. With the option -K
you store your passphrases in the keychain.
$ ssh-add -K ~/.ssh/id_rsa_github_user01
# here you have to enter your passphrases
$ ssh-add -K ~/.ssh/id_rsa_github_user02
# here you have to enter your passphrases
Check if the keys are successfully added.
$ ssh-add -l
4096 SHA256:O2MoTWlJD8yE+VxrxR5bOu0 ~/.ssh/id_rsa_github_user01 (RSA)
4096 SHA256:W2cnWtNK9tU9/dkWvOjLxNQ ~/.ssh/id_rsa_github_user02 (RSA)
3. Add your public ssh key to the GitHub
Add your public ssh key to the GitHub user 1
Copy your public key to your clipboard.
$ pbcopy < ~/.ssh/id_rsa_github_user01.pub
Login to your GitHub account user01. Go to https://github.com/settings/ssh/new, type in a title (e.g. id_rsa_github_user01), paste your key and click ‘Add SSH key’. Now you have to confirm with your GitHub password.
Add your public SSH key to the GitHub user 2
Copy your public key to your clipboard.
$ pbcopy < ~/.ssh/id_rsa_github_user02.pub
Login to your GitHub account user02. Go to https://github.com/settings/ssh/new, type in a title (e.g. id_rsa_github_user02), paste your key and click ‘Add SSH key’. Now you have to confirm with your GitHub password.
4. Preconfigure git
To prevent you from committing to a GitHub repository with a wrong committer email address or username, you should remove the email address and name from the global git config. These 2 configs, Email address and username, should be configured for each repository separately.
# Remove email address from global config:
$ git config --global --unset-all user.email
$ git config --global --unset-all user.name
# Require setting user.name and email per-repo:
$ git config --global user.useConfigOnly true
5. Add SSH keys to you git config
#GitHub account user01
Host id_rsa_github_user01
HostName github.com
User user01
PreferredAuthentications publickey
IdentityFile ~/.ssh/id_rsa_github_user01
#GitHub account user02
Host id_rsa_github_user02
HostName github.com
User user02
PreferredAuthentications publickey
IdentityFile ~/.ssh/github.com-work
6. Clone a GitHub repository
Every time you want to clone a repository from github, you have to replace github.com in the clone url with the corresponding host-id from your ~/ssh/config
.
Example:
# user01 wants to clone git@github.com:user01/testrepo01.git
$ git clone git@id_rsa_github_user01:user01/testrepo01.git
After a successful clone you have to configure the user.name and user.email of the repository.
$ git config user.name "John Doe"
$ git config user.email "john.doe@abc.com"